top of page
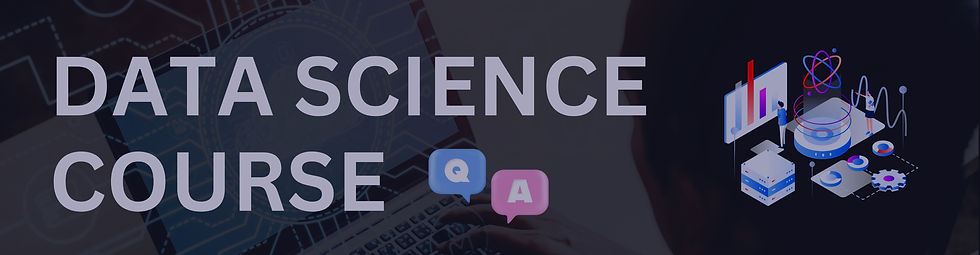
Data Science Interview Questions and Answers
Top 100 Data Science Interview Questions for Freshers
Data Science is one of the most in-demand skills in top tech companies, including IDM TechPark. Mastering both statistical analysis, machine learning, data visualization, databases, and deployment strategies makes a Data Scientist a valuable asset in modern software development. To secure a Data Science role at IDM TechPark, candidates must be proficient in technologies like Python, R, SQL, machine learning frameworks, data visualization tools, and cloud services, as well as be ready to tackle both the Data Science Online Assessment and Technical Interview Round.
To help you succeed, we have compiled a list of the Top 100 Data Science Interview Questions along with their answers. Mastering these will give you a strong edge in cracking Data Science interviews at IDM TechPark.
​1. Reverse a String
-
Question: Write a function to reverse a string without using built-in functions.
-
Answer:
def reverse_string(s): return s[::-1] # Example usage print(reverse_string("hello")) # Output: "olleh"
2. Find the Missing Number
-
Question: Given an array of n-1 integers from 1 to n, find the missing number.
-
Answer:
def find_missing_number(arr): n = len(arr) + 1 total_sum = n * (n + 1) // 2 return total_sum - sum(arr) # Example usage print(find_missing_number([1, 2, 4, 5, 6])) # Output: 3
3. Check for Palindrome
-
Question: Write a function to check if a string is a palindrome.
-
Answer:
def is_palindrome(s): return s == s[::-1] # Example usage print(is_palindrome("madam")) # Output: True
4. Find the Largest Element in an Array
-
Question: Write a function to find the largest element in an array.
-
Answer:
def find_largest(arr): return max(arr) # Example usage print(find_largest([3, 1, 4, 1, 5, 9])) # Output: 9
5. Fibonacci Sequence
-
Question: Write a function to generate the nth Fibonacci number.
-
Answer:
def fibonacci(n): a, b = 0, 1 for _ in range(n): a, b = b, a + b return a # Example usage print(fibonacci(7)) # Output: 13
6. Find the First Non-Repeating Character
-
Question: Given a string, find the first non-repeating character.
-
Answer:
def first_non_repeating_char(s): for char in s: if s.count(char) == 1: return char return None # Example usage print(first_non_repeating_char("swiss")) # Output: "w"
7. Count the Number of Vowels
-
Question: Write a function to count the number of vowels in a given string.
-
Answer:
def count_vowels(s): vowels = 'aeiou' return sum(1 for char in s if char in vowels) # Example usage print(count_vowels("hello")) # Output: 2
8. Find the Intersection of Two Arrays
-
Question: Write a function to find the intersection of two arrays.
-
Answer:
def intersection(arr1, arr2): return list(set(arr1) & set(arr2)) # Example usage print(intersection([1, 2, 2, 1], [2, 2])) # Output: [2]
9. Merge Sorted Arrays
-
Question: Merge two sorted arrays into one sorted array.
-
Answer:
def merge_sorted_arrays(arr1, arr2): return sorted(arr1 + arr2) # Example usage print(merge_sorted_arrays([1, 3, 5], [2, 4, 6])) # Output: [1, 2, 3, 4, 5, 6]
10. Find the Factorial of a Number
-
Question: Write a function to calculate the factorial of a number using recursion.
-
Answer:
def factorial(n): if n == 0: return 1 return n * factorial(n - 1) # Example usage print(factorial(5)) # Output: 120
11. Check if a Number is Prime
-
Question: Write a function to check if a number is prime.
-
Answer:
def is_prime(n): if n <= 1: return False for i in range(2, int(n**0.5) + 1): if n % i == 0: return False return True # Example usage print(is_prime(7)) # Output: True
12. Rotate an Array
-
Question: Rotate an array by k positions.
-
Answer:
def rotate_array(arr, k): return arr[-k % len(arr):] + arr[:-k % len(arr)] # Example usage print(rotate_array([1, 2, 3, 4, 5], 2)) # Output: [4, 5, 1, 2, 3]
13. Find Duplicate in an Array
-
Question: Write a function to find if there are any duplicates in an array.
-
Answer:
def has_duplicates(arr): return len(arr) != len(set(arr)) # Example usage print(has_duplicates([1, 2, 3, 4, 5])) # Output: False
14. Sum of Two Numbers
-
Question: Write a function to find if any two numbers in an array sum up to a target value.
-
Answer:
def two_sum(arr, target): seen = set() for num in arr: if target - num in seen: return True seen.add(num) return False # Example usage print(two_sum([1, 2, 3, 4], 7)) # Output: True
15. Count the Number of Words in a String
-
Question: Write a function to count the number of words in a string.
-
Answer:
def word_count(s): return len(s.split()) # Example usage print(word_count("Hello world!")) # Output: 2
16. Find the Longest Substring Without Repeating Characters
-
Question: Write a function to find the length of the longest substring without repeating characters.
-
Answer:
def longest_unique_substring(s): char_set = set() left = 0 max_len = 0 for right in range(len(s)): while s[right] in char_set: char_set.remove(s[left]) left += 1 char_set.add(s[right]) max_len = max(max_len, right - left + 1) return max_len # Example usage print(longest_unique_substring("abcabcbb")) # Output: 3
17. Binary Search
-
Question: Implement binary search in a sorted array.
-
Answer:
def binary_search(arr, target): left, right = 0, len(arr) - 1 while left <= right: mid = (left + right) // 2 if arr[mid] == target: return mid elif arr[mid] < target: left = mid + 1 else: right = mid - 1 return -1 # Example usage print(binary_search([1, 2, 3, 4, 5], 4)) # Output: 3
18. Count Inversions in an Array
-
Question: Write a function to count the number of inversions in an array (pair of elements where the first is greater than the second).
-
Answer:
def count_inversions(arr): def merge_count_split_inv(arr, temp_arr, left, right): if left == right: return 0 mid = (left + right) // 2 inv_count = merge_count_split_inv(arr, temp_arr, left, mid) inv_count += merge_count_split_inv(arr, temp_arr, mid + 1, right) inv_count += merge_and_count(arr, temp_arr, left, mid, right) return inv_count def merge_and_count(arr, temp_arr, left, mid, right): i = left # Starting index for left subarray j = mid + 1 # Starting index for right subarray k = left # Starting index to be sorted inv_count = 0 while i <= mid and j <= right: if arr[i] <= arr[j]: temp_arr[k] = arr[i] i += 1 else: temp_arr[k] = arr[j] inv_count += (mid-i + 1) j += 1 k += 1 while i <= mid: temp_arr[k] = arr[i] i += 1 k += 1 while j <= right: temp_arr[k] = arr[j] j += 1 k += 1 for i in range(left, right + 1): arr[i] = temp_arr[i] return inv_count temp_arr = [0] * len(arr) return merge_count_split_inv(arr, temp_arr, 0, len(arr) - 1) # Example usage print(count_inversions([1, 20, 6, 4, 5])) # Output: 5
19. Implement a Queue using Two Stacks
-
Question: Implement a queue using two stacks.
-
Answer:
class QueueUsingStacks: def __init__(self): self.stack1 = [] self.stack2 = [] def enqueue(self, item): self.stack1.append(item) def dequeue(self): if not self.stack2: while self.stack1: self.stack2.append(self.stack1.pop()) return self.stack2.pop() if self.stack2 else None q = QueueUsingStacks() q.enqueue(1) q.enqueue(2) print(q.dequeue()) # Output: 1
20. Implement a Stack using Queues
-
Question: Implement a stack using two queues.
-
Answer:
class StackUsingQueues: def __init__(self): self.queue1 = [] self.queue2 = [] def push(self, item): self.queue1.append(item) def pop(self): if len(self.queue1) == 0: return None while len(self.queue1) > 1: self.queue2.append(self.queue1.pop(0)) item = self.queue1.pop() self.queue1, self.queue2 = self.queue2, self.queue1 return item stack = StackUsingQueues() stack.push(1) stack.push(2) print(stack.pop()) # Output: 2
21. Find the Missing Number in an Array of Consecutive Numbers
-
Question: Write a function to find the missing number in a sequence of consecutive numbers.
-
Answer:
def find_missing(arr): total_sum = sum(range(arr[0], arr[-1] + 1)) return total_sum - sum(arr) # Example usage print(find_missing([1, 2, 4, 5, 6])) # Output: 3
22. Check if a String is an Anagram
-
Question: Write a function to check if two strings are anagrams.
-
Answer:
def is_anagram(s1, s2): return sorted(s1) == sorted(s2) # Example usage print(is_anagram("listen", "silent")) # Output: True
23. Longest Common Prefix
-
Question: Write a function to find the longest common prefix in an array of strings.
-
Answer:
def longest_common_prefix(strs): if not strs: return "" prefix = strs[0] for string in strs[1:]: while string[:len(prefix)] != prefix: prefix = prefix[:-1] if not prefix: return "" return prefix # Example usage print(longest_common_prefix(["flower", "flow", "flight"])) # Output: "fl"
24. Find the Kth Largest Element in an Array
-
Question: Find the Kth largest element in an unsorted array.
-
Answer:
import heapq def find_kth_largest(arr, k): return heapq.nlargest(k, arr)[-1] # Example usage print(find_kth_largest([3, 2, 1, 5, 6, 4], 2)) # Output: 5
25. Check if a String is a Valid Parenthesis Expression
-
Question: Write a function to check if a given string of parentheses is valid.
-
Answer:
def is_valid_parentheses(s): stack = [] mapping = {")": "(", "}": "{", "]": "["} for char in s: if char in mapping: top_element = stack.pop() if stack else '#' if mapping[char] != top_element: return False else: stack.append(char) return not stack # Example usage print(is_valid_parentheses("()[]{}")) # Output: True
bottom of page